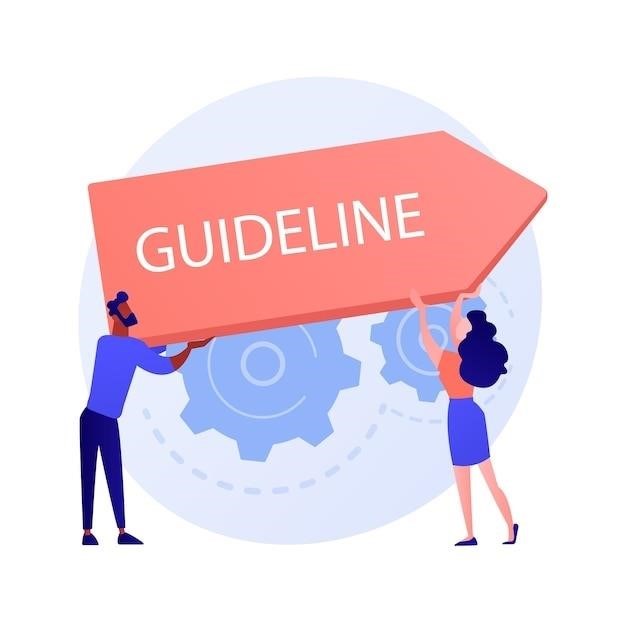
Understanding GUIDs
GUIDs, or Globally Unique Identifiers, are 128-bit values used to uniquely identify resources across different systems and networks. They are commonly used in software development, databases, and other applications where unique identification is crucial.
What is a GUID?
A GUID, or Globally Unique Identifier, is a 128-bit value used to uniquely identify resources across different systems and networks. It’s often referred to as a UUID (Universally Unique Identifier) as well. Think of a GUID like a digital fingerprint – it’s a unique identifier for a specific entity, ensuring it stands out from all other entities. GUIDs are commonly used in software development, databases, and other applications where unique identification is crucial. They guarantee that each entity has a distinct identity, preventing conflicts and ensuring proper data management.
GUID Format
GUIDs are typically represented as a 36-character string, divided into five groups separated by hyphens. This format ensures readability and helps differentiate GUIDs. The standard format is 8-4-4-4-12, representing the number of hexadecimal digits in each group. Each group consists of hexadecimal characters (0-9 and a-f), representing the 128 bits of the GUID. The first group represents the most significant bits, while the last group represents the least significant bits. This structured format allows for easy parsing and validation, making it efficient for various applications.
Uses of GUIDs
GUIDs are versatile and widely used in various domains, including software development, databases, and networking. Their primary purpose is to ensure unique identification, preventing conflicts and ensuring data integrity. In software development, GUIDs are often used to identify objects, components, or resources, ensuring that each element has a distinct identifier. Databases utilize GUIDs as primary keys, guaranteeing that each record has a unique identifier, simplifying data management and retrieval. Network applications leverage GUIDs for device identification, facilitating communication and resource allocation. The unique nature of GUIDs makes them suitable for distributed systems, preventing collisions even when generating IDs across different systems or networks.
Validating GUIDs with Regex
Regular expressions (regex) provide a powerful way to validate GUIDs, ensuring they conform to the expected format and structure.
The Regex Pattern
The regex pattern for validating GUIDs is designed to match the standard 8-4-4-4-12 hexadecimal format. This pattern is typically represented as⁚ ^{?([0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12})}$
. Let’s break down this pattern to understand its components.
The pattern starts with an optional opening curly brace ({?
), allowing for GUIDs that may or may not be enclosed in braces. It then defines a group ((...)
) to capture the main GUID string. This group consists of five parts⁚
[0-9a-fA-F]{8}
⁚ Matches eight hexadecimal characters.-[0-9a-fA-F]{4}
⁚ Matches a hyphen followed by four hexadecimal characters.-[0-9a-fA-F]{4}
⁚ Matches a hyphen followed by four hexadecimal characters.-[0-9a-fA-F]{4}
⁚ Matches a hyphen followed by four hexadecimal characters.-[0-9a-fA-F]{12}
⁚ Matches a hyphen followed by twelve hexadecimal characters.
Finally, the pattern ends with an optional closing curly brace (}
) to match GUIDs that may be enclosed in braces.
Breakdown of the Pattern
The regex pattern for validating GUIDs is designed to match the standard 8-4-4-4-12 hexadecimal format, ensuring that the string adheres to the expected structure of a GUID. Let’s break down the pattern to understand its components and their significance.
The pattern starts with an optional opening curly brace {?
, allowing for GUIDs that may or may not be enclosed in braces. It then defines a group (...)
to capture the main GUID string. This group consists of five parts, each representing a specific segment of the GUID structure⁚
[0-9a-fA-F]{8}
⁚ This part matches eight hexadecimal characters, representing the first segment of the GUID. Hexadecimal characters include digits 0-9 and letters A-F (both uppercase and lowercase).-[0-9a-fA-F]{4}
⁚ This part matches a hyphen followed by four hexadecimal characters. The hyphen acts as a separator between segments of the GUID, and the four hexadecimal characters represent the second segment of the GUID.-[0-9a-fA-F]{4}
⁚ This part matches another hyphen followed by four hexadecimal characters, representing the third segment of the GUID.-[0-9a-fA-F]{4}
⁚ This part matches yet another hyphen followed by four hexadecimal characters, representing the fourth segment of the GUID.-[0-9a-fA-F]{12}
⁚ This part matches a final hyphen followed by twelve hexadecimal characters, representing the fifth and final segment of the GUID.
Finally, the pattern ends with an optional closing curly brace }
to match GUIDs that may be enclosed in braces.
Example Implementation
Let’s illustrate how to use the regex pattern for GUID validation in a practical scenario. Imagine you are developing a web application that requires users to input GUIDs. You want to ensure that the input is in the correct format before processing it further. Here’s a simple JavaScript example using the regex pattern⁚
function isValidGuid(guid) {
const regex = /^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$/;
return regex.test(guid);
}
const guid1 = '12345678-1234-1234-1234-1234567890AB';
const guid2 = '123456781234123412341234567890AB';
console.log(`Is ${guid1} a valid GUID? ${isValidGuid(guid1)}`);
console.log(`Is ${guid2} a valid GUID? ${isValidGuid(guid2)}`);
In this code, the isValidGuid
function takes a string as input and uses the regex pattern to check if it matches the GUID format. The function returns true
if the input is a valid GUID and false
otherwise. The example then tests two GUIDs, one valid and one invalid, demonstrating how the regex pattern can be used to validate GUID input.
GUID Validation in Programming Languages
Validating GUIDs is a common task in various programming languages. This section explores how to achieve this using regular expressions and specific language libraries.
JavaScript
JavaScript provides a straightforward way to validate GUIDs using regular expressions. The following code snippet demonstrates a function that checks if a string is a valid GUID using the standard format⁚
function isValidGuid(guid) {
const regex = /^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$/;
return regex.test(guid);
}
This function defines a regular expression that matches the expected GUID format. It checks for 32 hexadecimal characters separated by hyphens in the standard 8-4-4-4-12 pattern. The test
method returns true if the provided guid
string matches the regular expression, indicating a valid GUID.
Alternatively, JavaScript offers a built-in Guid
object that can be used for validation. The following code demonstrates how to use this object⁚
function isValidGuid(guid) {
try {
new Guid(guid);
return true;
} catch (error) {
return false;
}
}
This function attempts to create a new Guid
object from the provided guid
string. If successful, it returns true, indicating a valid GUID. If an exception is thrown during object creation, it returns false, indicating an invalid GUID.
Python
Python offers a simple and efficient way to validate GUIDs using regular expressions. The re
module provides the necessary tools for pattern matching. The following code snippet demonstrates a function that checks if a string is a valid GUID using the standard format⁚
import re
def is_valid_guid(guid)⁚
regex = re.compile(r'^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$')
match = regex.match(guid)
return bool(match)
This function utilizes a regular expression pattern that matches the expected GUID format. It checks for 32 hexadecimal characters separated by hyphens in the standard 8-4-4-4-12 pattern. The match
method returns a match object if the provided guid
string matches the regular expression, indicating a valid GUID. The bool
function converts the match object to a boolean value, returning true if a match was found, and false otherwise.
Alternatively, Python’s uuid
module provides a built-in class for working with GUIDs. The following code demonstrates how to use this module for validation⁚
import uuid
def is_valid_guid(guid)⁚
try⁚
uuid.UUID(guid)
return True
except ValueError⁚
return False
This function attempts to create a new UUID
object from the provided guid
string. If successful, it returns true, indicating a valid GUID. If a ValueError
exception is raised during object creation, it returns false, indicating an invalid GUID.
Java
Java provides a robust mechanism for validating GUIDs using regular expressions. The java.util.regex
package offers classes for pattern matching and string manipulation. Here’s a Java code snippet demonstrating a function to validate GUIDs⁚
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class GUIDValidator {
public static boolean isValidGUID(String guid) {
String regex = "^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(guid);
return matcher.matches;
}
public static void main(String[] args) {
String validGUID = "12345678-1234-1234-1234-1234567890AB";
String invalidGUID = "12345678-1234-1234-1234-1234567890ABC";
System.out.println(validGUID + "⁚ " + isValidGUID(validGUID));
System.out.println(invalidGUID + "⁚ " + isValidGUID(invalidGUID));
}
}
This code defines a function isValidGUID
that takes a guid
string as input. It uses a regular expression pattern matching the standard GUID format. The Pattern.compile
method compiles the regex into a Pattern
object, while pattern.matcher
creates a Matcher
object for the input string. The matcher.matches
method returns true if the input matches the pattern, indicating a valid GUID, and false otherwise.
The main
method demonstrates the usage of the validation function with a valid and an invalid GUID string, printing the results to the console.
C#
C#, a popular language for .NET development, offers a built-in Guid
structure for representing GUIDs. While using the Guid.TryParse
method is often the most efficient way to validate a GUID, you can still utilize regular expressions for this purpose. Here’s a C# code example demonstrating GUID validation with regex⁚
using System;
using System.Text.RegularExpressions;
public class GUIDValidator {
public static bool IsValidGUID(string guid) {
string pattern = @"^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$";
return Regex.IsMatch(guid, pattern);
}
public static void Main(string[] args) {
string validGUID = "12345678-1234-1234-1234-1234567890AB";
string invalidGUID = "12345678-1234-1234-1234-1234567890ABC";
Console.WriteLine(validGUID + "⁚ " + IsValidGUID(validGUID));
Console.WriteLine(invalidGUID + "⁚ " + IsValidGUID(invalidGUID));
}
}
This code defines a function IsValidGUID
that takes a guid
string as input. It uses a regular expression pattern matching the standard GUID format. The Regex.IsMatch
method checks if the input string matches the pattern. If it does, it returns true, indicating a valid GUID, otherwise it returns false.
The Main
method demonstrates the usage of the validation function with a valid and an invalid GUID string, printing the results to the console.
PHP
PHP, a widely used server-side scripting language, also provides functionality for working with GUIDs. While PHP offers built-in functions like uniqid
for generating unique identifiers, you can leverage regular expressions for validating GUIDs. Here’s an example of how to implement GUID validation with regex in PHP⁚
<?php
function is_valid_guid($guid) {
$pattern = "/^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$/";
return preg_match($pattern, $guid) === 1;
}
$valid_guid = "12345678-1234-1234-1234-1234567890AB";
$invalid_guid = "12345678-1234-1234-1234-1234567890ABC";
echo $valid_guid . "⁚ " . (is_valid_guid($valid_guid) ? "Valid" ⁚ "Invalid") . "<br>";
echo $invalid_guid . "⁚ " . (is_valid_guid($invalid_guid) ? "Valid" ⁚ "Invalid") . "<br>";
?>
This PHP code defines a function is_valid_guid
that takes a $guid
string as input. It uses a regular expression pattern matching the standard GUID format. The preg_match
function checks if the input string matches the pattern. If it does, it returns 1, indicating a valid GUID, otherwise it returns 0.
The code demonstrates the usage of the validation function with a valid and an invalid GUID string, printing the results.
Additional Considerations
While regex provides a powerful tool for validating GUIDs, it’s crucial to consider other aspects related to GUID generation, storage, and best practices.
GUID Generation
While regex focuses on validating existing GUIDs, understanding how they are generated is essential for proper usage. Most programming languages provide built-in functions for generating GUIDs, often leveraging algorithms like version 4 UUIDs. These algorithms ensure randomness and minimize the chance of collisions, making them suitable for various applications.
Generating GUIDs involves using random number generators, ensuring a unique identifier for each instance. However, it is crucial to note that GUID generation algorithms are designed to minimize, not eliminate, the possibility of collisions. Therefore, while the likelihood of two identical GUIDs is statistically improbable, it is not impossible. For critical applications where absolute uniqueness is paramount, consider incorporating additional safeguards like collision detection mechanisms or using alternative unique identification methods.
GUID in Databases
Databases often employ GUIDs as primary keys for tables, ensuring uniqueness and avoiding conflicts when managing data. This is particularly beneficial for distributed systems where data is spread across multiple servers. Using GUIDs as primary keys eliminates the need for complex synchronization mechanisms, simplifying data management and reducing potential errors.
However, storing GUIDs in databases can introduce performance considerations. The length of GUIDs can impact query performance, especially when using index-based searches. Strategies like optimizing database indexing and leveraging appropriate data types can mitigate these performance concerns. Additionally, consider the impact of GUID length on storage requirements, especially in databases with large datasets. For scenarios where performance is critical, explore alternative approaches like using shorter unique identifiers or optimizing database design to minimize the impact of GUIDs.
GUID Best Practices
While GUIDs provide a robust solution for unique identification, adhering to best practices ensures their effective and efficient utilization. Prioritize using well-established libraries and tools for generating GUIDs to guarantee their authenticity and randomness. This minimizes the risk of collisions and ensures the integrity of your unique identifiers.
Store and handle GUIDs consistently, using a consistent format and representation throughout your application. This simplifies data management, improves interoperability, and reduces the likelihood of errors. Avoid modifying or manipulating GUIDs after generation, as any alterations can compromise their uniqueness and introduce inconsistencies.
Regularly review your GUID implementation, particularly in high-volume or critical systems, to ensure its continued effectiveness and address any potential performance bottlenecks. By following these best practices, you can maximize the benefits of GUIDs while mitigating potential issues, ensuring a reliable and efficient system.
Resources and Tools
Leveraging online resources and tools can significantly aid in working with GUIDs and regex patterns.
Regex Testers
Regex testers are invaluable tools for developers and anyone working with regular expressions. They provide an interactive environment to experiment with different patterns, test their effectiveness, and analyze their behavior against various input strings. Online regex testers often offer features like syntax highlighting, real-time matching, and detailed explanations of the regex pattern’s components.
Popular regex testers include⁚
- regex101⁚ This widely used tool provides a user-friendly interface for creating, testing, and debugging regex patterns. It offers features like syntax highlighting, pattern explanation, and support for multiple programming languages.
- Regexr⁚ Another excellent choice, Regexr provides a similar set of features as regex101, including support for various regex flavors, pattern visualization, and a cheat sheet for commonly used regex constructs.
- Debuggex⁚ This online tester distinguishes itself with its visual representation of regex patterns, making it easier to understand the logic behind complex expressions. It also offers features like highlighting matches and generating code in different programming languages.
By utilizing these online regex testers, developers can quickly validate and refine their GUID validation regex patterns, ensuring accurate and reliable results.
GUID Generators
GUID generators are essential tools for developers who need to create unique identifiers for various purposes. They automate the process of generating valid GUIDs, ensuring that each identifier is distinct and globally unique. These generators typically provide options for customizing the format of the generated GUID, allowing users to specify the desired length, character set, and other parameters.
Many online and offline GUID generators are available. Some popular options include⁚
- GUID Generator (Online)⁚ This website offers a simple yet effective tool for generating both version 1 and version 4 GUIDs. It also provides a clear display of the generated GUID and allows users to copy it for easy integration into their applications.
- UUID Generator (Online)⁚ This online tool specializes in generating UUIDs (Universally Unique Identifiers), which are essentially the same as GUIDs. It offers various customization options and provides the generated UUID in different formats, including string, hexadecimal, and binary.
- Microsoft GUID Generator⁚ For users working within the Microsoft ecosystem, the GUID generator integrated into Visual Studio provides a convenient way to create GUIDs directly within their development environment.
These generators streamline the process of creating unique identifiers, enabling developers to focus on the core logic of their applications without worrying about generating valid and unique GUIDs.